EJB stands for Enterprise Java Bean. If you are not fimilar with EJB, Please read below in order to move ahead with "Creating EJB 3.1 Application using WebLogic 12.1.1".
EJB application requires to be run in Application Server like WebLogic, JBoss. As part of current EJB Demo, WebLogic Server 12.1.1 and JDK 1.7 Update 03 is used.
Suppose, I want to write Stateless Session Bean(Can be local or remote), in My case Remote Component which will provide customer Information upon request from Client.
EJB 3.1 Remote Interface for Session Bean Example using Weblogic Server 12.1.1:
Create Remote Interface : CustomerServiceRemote.java
package com.anuj.business; import javax.ejb.Remote; import com.anuj.business.rep.Customer; @Remote public interface CustomerServiceRemote { public Customer getCustomerInfo(String customerId); }
Create Session bean implementation Class : CustomerService.java
package com.anuj.business; import java.util.ArrayList; import java.util.List; import javax.ejb.Stateless; import com.anuj.business.rep.Customer; /** * Session Bean implementation class CustomerService */ @Stateless(mappedName="customerServiceEJB") public class CustomerService implements CustomerServiceRemote { private List customers = new ArrayList(); /** * Default constructor. */ public CustomerService() { createCustomers(); } @Override public Customer getCustomerInfo(String customerId) { Customer customer = null; for(Customer cust : customers){ if(cust.getCustomerId().equals(customerId)){ customer = cust; break; } } if(customer != null){ return customer; } else{ return null; } } protected void createCustomers(){ Customer customer = new Customer(); customer.setCustomerId("1"); customer.setCustomerName("Anuj"); customer.setAddress("India"); customer.setPhone("1234567890"); customers.add(customer); } }
Deploy EJB to WebLogic Server :
Now, you have Session Bean available. Export EJB jar and Deploy it to WebLogic Server.
Create Client :
Creating Client which will access Remote EJB deployed on Server, includes following steps
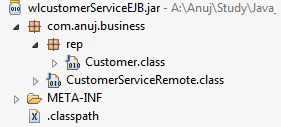
3. Configure Properties for WebLogic
Properties properties = new Properties(); properties.put(Context.INITIAL_CONTEXT_FACTORY,"weblogic.jndi.WLInitialContextFactory"); properties.put(Context.SECURITY_PRINCIPAL,"weblogic"); properties.put(Context.SECURITY_CREDENTIALS,"weblogic1"); properties.put(Context.PROVIDER_URL,"t3://localhost:7001");
4. Initialize Context with Configured Properties which will be used for JNDI Lookup
import javax.naming.InitialContext; Context context = new InitialContext(properties);
5. Perform JNDI lookup using Context. customerServiceEJB used below is MappedName which we have defined in CustomerSessionBean EJb Earlier.
context.lookup("customerServiceEJB#com.anuj.business.CustomerServiceRemote");
6. Access Remote Interface method
Customer customer = customerService.getCustomerInfo("1"); System.out.println("Customer Id : "+customer.getCustomerId()); System.out.println("Customer Name : "+customer.getCustomerName()); System.out.println("Address : " + customer.getAddress()); System.out.println("Phone : " + customer.getPhone());
Complete EJB 3.1 SessionBean example with Remote Interface using WebLogic Server 12c
import java.util.Properties; import javax.naming.Context; import javax.naming.InitialContext; import javax.naming.NamingException; import com.anuj.business.CustomerServiceRemote; import com.anuj.business.rep.Customer; public class CustomerServiceEJBClient { /** * @param args */ public static void main(String[] args) { Properties properties = new Properties(); properties.put(Context.INITIAL_CONTEXT_FACTORY,"weblogic.jndi.WLInitialContextFactory"); properties.put(Context.SECURITY_PRINCIPAL,"weblogic"); properties.put(Context.SECURITY_CREDENTIALS,"weblogic1"); properties.put(Context.PROVIDER_URL,"t3://localhost:7001"); try { Context context = new InitialContext(properties); CustomerServiceRemote customerService = (CustomerServiceRemote)context.lookup("customerServiceEJB#com.anuj.business.CustomerServiceRemote"); Customer customer = customerService.getCustomerInfo("1"); System.out.println("Customer Id : "+customer.getCustomerId()); System.out.println("Customer Name : "+customer.getCustomerName()); System.out.println("Address : " + customer.getAddress()); System.out.println("Phone : " + customer.getPhone()); } catch (NamingException e) { e.printStackTrace(); } } }
Output :
Customer Id : 1 Customer Name : Anuj Address : India Phone : 1234567890
After a lots of efforts I finally made my EJB Example up and Running. Hope this tutorial helps you for understanding EJB.
if My Tutorials helped you in any case then Like it or provide your feedback. You know, What? providing feedback or comments or like makes blogger happy:)
Hi, Very good Article.Can you pls tell me how to call local bean?
ReplyDeleteSimple, but I can understand what I am looking or. Thank you
ReplyDelete